Basit Windows Uygulamaları
Sinan Arslan
02:44
C#
,
Harf Yakalama Oyunu
,
Sayı Bulmaca
,
Slot Makinası Oyunu
,
Windows Forms
,
Windows Forms At Yarışı Oyunu
,
Windows Forms Sayısal Loto Oyunu
Hiç yorum yok
C # Sayı Bulmaca Oyunu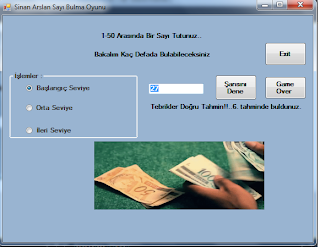
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; namespace SinanArslanSayiBulmaca { public partial class Form1 : Form { public Form1() { InitializeComponent(); } Random rnd = new Random(); int sayi; int sayac = 0; int hak; private void Form1_Load(object sender, EventArgs e) { this.Opacity = 0; timer1.Interval = 10; timer1.Enabled = true; this.Height = 266; pictureBox1.Visible = false; sayi = rnd.Next(1, 50); } private void timer1_Tick(object sender, EventArgs e) { this.Opacity = this.Opacity + 0.005; if (this.Opacity == 1) { timer1.Enabled = false; } } private void Form1_FormClosing(object sender, FormClosingEventArgs e) { if (KapatTamam == true) { e.Cancel = false; } else { e.Cancel = true; timer1.Enabled = false; timer2.Interval = 10; timer2.Enabled = true; } } bool KapatTamam = false; private void timer2_Tick(object sender, EventArgs e) { this.Opacity = this.Opacity - 0.005; if (this.Opacity == 0) { KapatTamam = true; this.Close(); } } private void btnDene_Click(object sender, EventArgs e) { if (rbbaslangic.Checked == true) { hak = 10; } else if (rbOrta.Checked == true) { hak = 7; } else if (rbileri.Checked == true) { hak = 4; } sayac++; if (sayac < hak) { int tahmin = Convert.ToInt32(txttahmin.Text); if (tahmin < sayi) { label3.Text = "Tahmininz Küçük"; } else if (tahmin > sayi) { label3.Text = "Tahmininiz Büyük.."; } else { label3.Text = "Tebrikler Doğru Tahmin!!.." + sayac + ". tahminde buldunuz."; pictureBox1.Visible = true; } txttahmin.Focus(); txttahmin.Select(0, 2); this.Height = 450; } else { label3.Text = "Hakkınızı kaybettiniz"; btnDene.Enabled = false; } } private void btnCikis_Click(object sender, EventArgs e) { this.Close(); } private void btnOyunSonu_Click(object sender, EventArgs e) { btnDene.Enabled = true; sayi = rnd.Next(1, 50); this.Height = 405; sayac = 0; txttahmin.Text = ""; txttahmin.Focus(); txttahmin.Select(0, 2); label3.Text = ""; pictureBox1.Visible = false; } } }
================================================== ====================
C # Harf Yakalama Oyunu
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; namespace _23_HarfYakalamaca { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { this.Width = 900; this.Height = 700; this.BackColor = Color.White; timer1.Interval = 2001; timer1.Enabled = true; } Random rnd = new Random(); private void timer1_Tick(object sender, EventArgs e) { /* * 1. Kordinat için soldan ve yukardan mesafe * 2. Harf Goster */ this.BackColor = Color.White; int Soldan = rnd.Next(0, this.Width - 50); int Yukardan = rnd.Next(0, this.Height - 50); label1.Left = Soldan; label1.Top = Yukardan; int AsciiKodu = rnd.Next(65, 91); //65 char KarakterYap = Convert.ToChar(AsciiKodu); //65 'A' label1.Text = KarakterYap.ToString(); //"A" } int DogruSayisi = 0; int YanlisSayisi = 0; private void Form1_KeyPress(object sender, KeyPressEventArgs e) { //eger (Klavyeden GElen == labeldan gelen) if (e.KeyChar.ToString() == label1.Text) { //AYNI this.BackColor = Color.Green; DogruSayisi++; if (timer1.Interval != 1) { //timer1.Interval -= 250; timer1.Interval = timer1.Interval - 250; } } else { //FARKLI YanlisSayisi++; this.BackColor = Color.Red; } this.Text = "Doğru Sayisi: " + DogruSayisi.ToString() + " - Yanlış Sayisi: " + YanlisSayisi.ToString() + " - Hız: " + timer1.Interval.ToString(); } } }
================================================== ======================
C # Slot Makinasi
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; namespace _12_SlotMakinasi { public partial class Form1 : Form { public Form1() { InitializeComponent(); } Random rnd = new Random(); int Sayac = 0; private void btnKendimiSansliHissediyorum_Click(object sender, EventArgs e) { Sayac = 0; timer1.Interval = 10; timer1.Enabled = true; } private void timer1_Tick(object sender, EventArgs e) { int Resim01 = rnd.Next(1, 6); int Resim02 = rnd.Next(1, 6); int Resim03 = rnd.Next(1, 6); #region Ilk Resim Secme Yeri if (Resim01 == 1) { pictureBox1.Image = Properties.Resources._001; } else if (Resim01 == 2) { pictureBox1.Image = Properties.Resources._002; } else if (Resim01 == 3) { pictureBox1.Image = Properties.Resources._003; } else if (Resim01 == 4) { pictureBox1.Image = Properties.Resources._004; } else if (Resim01 == 5) { pictureBox1.Image = Properties.Resources._005; } #endregion #region Ikinci Resim Secme Yeri if (Resim02 == 1) { pictureBox2.Image = Properties.Resources._001; } else if (Resim02 == 2) { pictureBox2.Image = Properties.Resources._002; } else if (Resim02 == 3) { pictureBox2.Image = Properties.Resources._003; } else if (Resim02 == 4) { pictureBox2.Image = Properties.Resources._004; } else if (Resim02 == 5) { pictureBox2.Image = Properties.Resources._005; } #endregion #region Üçüncü Resim Secme Yeri if (Resim03 == 1) { pictureBox3.Image = Properties.Resources._001; } else if (Resim03 == 2) { pictureBox3.Image = Properties.Resources._002; } else if (Resim03 == 3) { pictureBox3.Image = Properties.Resources._003; } else if (Resim03 == 4) { pictureBox3.Image = Properties.Resources._004; } else if (Resim03 == 5) { pictureBox3.Image = Properties.Resources._005; } #endregion Sayac++; //Sayac = Sayac + 1; //Sayac += 1; if (Sayac == 50) { timer1.Interval = 100; } else if (Sayac == 70) { timer1.Interval = 250; } else if (Sayac == 75) { timer1.Interval = 400; } else if (Sayac == 78) { timer1.Enabled = false; if (Resim01 == Resim02 && Resim01 == Resim03) { MessageBox.Show("Kazandiniz"); } else { MessageBox.Show("Kaybettin"); } } } } }================================================== ======================C# At Yarışı Oyunu using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; namespace _22_AtYarisi { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void btnBasla_Click(object sender, EventArgs e) { pbAt01.Left = 29; pbAt02.Left = 29; pbAt03.Left = 29; timer1.Interval = 40; timer1.Enabled = true; } Random rnd = new Random(); private void timer1_Tick(object sender, EventArgs e) { int at01Ilerleme = rnd.Next(1, 10); int at02Ilerleme = rnd.Next(1, 10); int at03Ilerleme = rnd.Next(1, 10); pbAt01.Left += at01Ilerleme; pbAt02.Left += at02Ilerleme; pbAt03.Left += at03Ilerleme; if (pbBitis.Left <= pbAt01.Right || pbBitis.Left <= pbAt02.Right || pbBitis.Left <= pbAt03.Right) { timer1.Enabled = false; } } private void button2_Click(object sender, EventArgs e) { } } } ========================================================================
C# Şanslı Kişi Seçme Oyunu
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; namespace _20_SanliKisiSecici { public partial class Form1 : Form { public Form1() { InitializeComponent(); } string[] Isimler; private void button1_Click(object sender, EventArgs e) { Isimler = new string[8]; Isimler[0] = "Ahmet"; Isimler[1] = "Mehmet"; Isimler[2] = "Kemal"; Isimler[3] = "Haydar"; Isimler[4] = "Nazan"; Isimler[5] = "Baki"; Isimler[6] = "Ali"; Isimler[7] = "Aslı"; Sayac = 0; label1.ForeColor = Color.Black; timer1.Interval = 1; timer1.Enabled = true; } Random rnd = new Random(); int Sayac = 0; private void timer1_Tick(object sender, EventArgs e) { int RastgeleSayi = rnd.Next(0, Isimler.Length); string RastgeleIsim = Isimler[RastgeleSayi]; label1.Text = RastgeleIsim; RastgeleSayi = rnd.Next(0, Isimler.Length); RastgeleIsim = Isimler[RastgeleSayi]; label2.Text = RastgeleIsim; Sayac++; switch (Sayac) { case 50: timer1.Interval = 50; break; case 60: timer1.Interval = 100; break; case 70: timer1.Interval = 130; break; case 80: timer1.Interval = 200; break; case 90: timer1.Interval = 350; break; case 100: timer1.Interval = 550; break; case 103: timer1.Interval = 700; break; case 105: timer1.Interval = 1100; break; case 113: timer1.Enabled = false; label1.ForeColor = Color.Red; if (label1.Text == label2.Text) { while (true) { RastgeleSayi = rnd.Next(0, Isimler.Length); RastgeleIsim = Isimler[RastgeleSayi]; label2.Text = RastgeleIsim; if (label1.Text != label2.Text) { break; } } } break; } } } } ====================================================================
C# Sayısal Loto Oyunu
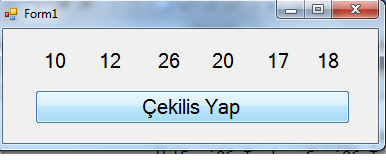
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; namespace _19_SayisalLoto2 { public partial class Form1 : Form { public Form1() { InitializeComponent(); } int[] Sayilar; int Sayac = 0; Random rnd = new Random(); private void btnCekilisYap_Click(object sender, EventArgs e) { Sayilar = new int[6]; int KucukSayac = 0; while (KucukSayac < 6) { int RastgeleSayi = rnd.Next(1, 50); //1..49 bool Varmi = Sayilar.Contains(RastgeleSayi); if (Varmi == false) { Sayilar[KucukSayac] = RastgeleSayi; KucukSayac++; } } //Array.Sort(Sayilar); timer1.Interval = 1; timer1.Enabled = true; } private void timer1_Tick(object sender, EventArgs e) { int Sayi01 = rnd.Next(1, 50); int Sayi02 = rnd.Next(1, 50); int Sayi03 = rnd.Next(1, 50); int Sayi04 = rnd.Next(1, 50); int Sayi05 = rnd.Next(1, 50); int Sayi06 = rnd.Next(1, 50); Sayac++; if (Sayac > 99 && Sayac < 120) { lblSayi01.Text = Sayilar[0].ToString(); lblSayi02.Text = Sayi02.ToString(); lblSayi03.Text = Sayi03.ToString(); lblSayi04.Text = Sayi04.ToString(); lblSayi05.Text = Sayi05.ToString(); lblSayi06.Text = Sayi06.ToString(); timer1.Interval = 100; } else if (Sayac > 119 && Sayac < 130) { lblSayi02.Text = Sayilar[1].ToString(); lblSayi03.Text = Sayi03.ToString(); lblSayi04.Text = Sayi04.ToString(); lblSayi05.Text = Sayi05.ToString(); lblSayi06.Text = Sayi06.ToString(); timer1.Interval = 200; } else if (Sayac > 129 && Sayac < 140) { lblSayi03.Text = Sayilar[2].ToString(); lblSayi04.Text = Sayi04.ToString(); lblSayi05.Text = Sayi05.ToString(); lblSayi06.Text = Sayi06.ToString(); timer1.Interval = 250; } else if (Sayac > 139 && Sayac < 150) { lblSayi04.Text = Sayilar[3].ToString(); lblSayi05.Text = Sayi05.ToString(); lblSayi06.Text = Sayi06.ToString(); timer1.Interval = 300; } else if (Sayac > 149 && Sayac < 160) { lblSayi05.Text = Sayilar[4].ToString(); lblSayi06.Text = Sayi06.ToString(); timer1.Interval = 400; } else if (Sayac == 160) { lblSayi06.Text = Sayilar[5].ToString(); timer1.Interval = 1; timer1.Enabled = false; Sayac = 0; } else { lblSayi01.Text = Sayi01.ToString(); lblSayi02.Text = Sayi02.ToString(); lblSayi03.Text = Sayi03.ToString(); lblSayi04.Text = Sayi04.ToString(); lblSayi05.Text = Sayi05.ToString(); lblSayi06.Text = Sayi06.ToString(); } } } }
Kaydol:
Kayıt Yorumları
(
Atom
)
Hiç yorum yok :
Yorum Gönder
Soru Görüş önerileriniz için gmail plus üzerinden + layın müsait olduğumda mutlaka yanıt dönüyor olacağım.